alglib::heap::binary_heap< elt_t, key_t > Class Template Reference
#include <binary_heap.h>
Inheritance diagram for alglib::heap::binary_heap< elt_t, key_t >:
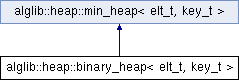
Public Types | |
typedef elt_t | elt_type |
typedef key_t | key_type |
Public Member Functions | |
binary_heap () | |
template<typename InputIter > | |
binary_heap (InputIter elt_first, InputIter elt_last, InputIter key_first) | |
void | insert (const elt_t &elt, const key_t &key) |
const elt_t & | get_min_elt () const |
const key_t & | get_min_key () const |
void | delete_min () |
void | replace (const elt_t &elt, const key_t &key) |
int | size () const override |
bool | empty () const override |
void | update_key (const elt_t &elt, const key_t &key) |
Member Typedef Documentation
template<typename elt_t, typename key_t>
typedef elt_t alglib::heap::binary_heap< elt_t, key_t >::elt_type |
template<typename elt_t, typename key_t>
typedef key_t alglib::heap::binary_heap< elt_t, key_t >::key_type |
Constructor & Destructor Documentation
template<typename elt_t , typename key_t >
alglib::heap::binary_heap< elt_t, key_t >::binary_heap | ( | ) |
template<typename elt_t , typename key_t >
template<typename InputIter >
|
explicit |
Member Function Documentation
template<typename elt_t , typename key_t >
|
virtual |
Implements alglib::heap::min_heap< elt_t, key_t >.
template<typename elt_t, typename key_t>
|
inlineoverridevirtual |
Implements alglib::heap::min_heap< elt_t, key_t >.
template<typename elt_t , typename key_t >
|
virtual |
Implements alglib::heap::min_heap< elt_t, key_t >.
template<typename elt_t , typename key_t >
|
virtual |
Implements alglib::heap::min_heap< elt_t, key_t >.
template<typename elt_t, typename key_t>
|
virtual |
Implements alglib::heap::min_heap< elt_t, key_t >.
template<typename elt_t, typename key_t>
|
virtual |
Implements alglib::heap::min_heap< elt_t, key_t >.
template<typename elt_t, typename key_t>
|
inlineoverridevirtual |
Implements alglib::heap::min_heap< elt_t, key_t >.
template<typename elt_t, typename key_t>
|
virtual |
Implements alglib::heap::min_heap< elt_t, key_t >.
The documentation for this class was generated from the following file:
- alglib/heap/binary_heap.h